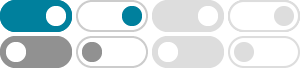
c - How does strchr implementation work - Stack Overflow
Jan 17, 2013 · const char * strchr ( const char * str, int character ); char * strchr ( char * str, int character ); Of course C can't do this. An alternative would have been to replace strchr by two functions, one taking a const char* and returning a const char*, and another taking a char* and returning a char*.
Confusion on how to use strchr in C - Stack Overflow
Jan 25, 2020 · char *strchr( const char *s, int c ); I understand that strchr locates the first occurrence of character c in string s. If c is found, a pointer to c in s is returned. Otherwise, a NULL pointer is
c - Why there is no strnchr function? - Stack Overflow
Jun 28, 2014 · Note that memchr is not exacly what one might expect strnchr might do, since memchr does not stop at the end of the string if it is shorter than the n argument (i.e. it can read bytes beyond the \0, unlike strchr). The "n-less" equivalent of memchr is not strchr, but rawmemchr, a GNU extension that is not POSIX nor C99. –
How would I replace the character in this example using strchr?
Sep 23, 2013 · char *chngChar (char *str, char oldChar, char newChar) { char *strPtr = str; while ((strPtr = strchr (strPtr, oldChar)) != NULL) *strPtr++ = newChar; return str; } It simply runs through the string looking for the specific character and replaces it with the new character.
find = strchr (st, '\n'); replaced with *find = '\0'; - Stack Overflow
Oct 18, 2018 · The code using find = strchr(s, '\n') and what follows zaps the newline that was read by fgets() and included in the result string, if there is indeed a newline in the string. Often, you can use an alternative, more compact, notation:
c - differences between memchr() and strchr() - Stack Overflow
Nov 23, 2010 · Differences can popup if you attempt to use a char* which stores binary data with strchr as it potentially won't see the full length of the string. This is true of pretty much any char* with binary data and a str* function. For non-binary data though they are virtually the same function. You can actually code up strchr in terms of memchr fairly ...
php - Difference between strstr() and strchr() - Stack Overflow
Mar 6, 2017 · You used strstr() which is an alias of strchr(), that's why it is just the same. But your reference compares strchr() and strrchr(), that's why you get a different result than from your reference. EDIT. Since you've edited your question. This is now my answer: You used strstr() which is just an alias of strchr(), that's why it is just the same.
string - how to get substring in c with strchr - Stack Overflow
Dec 23, 2015 · pose=strchr(mystring+b+1,' '); pose=strchr(&mystring[b+1],' '); The variables b and e should contain the positions of the space character in mystring. word2 should contain quick eventually. Another solution would be to 'walk through' the string using a loop, but that would be cheating the strchr function.
Comparing character with Intel x86_64 assembly - Stack Overflow
Mar 15, 2016 · asm_strchr: push rbp mov rbp, rsp strchr_loop: cmp byte [rdi], 0 ; test end of string je fail_end ; go to end cmp byte [rdi], sil ; check if current character is equal to character given in parameter je strchr_end ; go to end inc rdi ; move to next character of the string jmp strchr_loop ; loop strchr_end: mov rax, rdi ; set function return mov ...
How to use strchr() multiple times to find the nth occurrence
I am trying to figure out a way to make this work: //Returns a pointer to the nth char in a string const char *nth_strchr(const char *s, int c, int n) { int c_count = 0; char *nth_ptr;