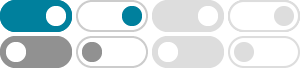
Implementation of Hash Table in C/C++ using Separate Chaining
Mar 1, 2023 · A hash table is a data structure that allows for quick insertion, deletion, and retrieval of data. It works by using a hash function to map a key to an index in an array. In this article, we will implement a hash table in Python using separate chaining to …
How to implement a hash table (in C) - Ben Hoyt
An explanation of how to implement a simple hash table data structure, with code and examples in the C programming language.
Hash Table Data Structure - GeeksforGeeks
Sep 15, 2024 · What is Hash Table? A Hash table is defined as a data structure used to insert, look up, and remove key-value pairs quickly. It operates on the hashing concept, where each key is translated by a hash function into a distinct index in an array. The index functions as a storage location for the matching value.
How To Implement a Sample Hash Table in C/C++ - DigitalOcean
Jan 13, 2023 · A hash table in C/C++ is a data structure that maps keys to values. A hash table uses a hash function to compute indexes for a key. You can store the value at the appropriate location based on the hash table index.
c - hash function for string - Stack Overflow
I'm working on hash table in C language and I'm testing hash function for string. The first function I've tried is to add ascii code and use modulo (% 100) but i've got poor results with the first ...
std::hash - cppreference.com
Jan 2, 2025 · std:: hash < const char * > produces a hash of the value of the pointer (the memory address), it does not examine the contents of any character array. Additional specializations for std::pair and the standard container types, as well as utility functions to compose hashes are available in boost::hash .
Program for hashing with chaining - GeeksforGeeks
Sep 15, 2024 · In hashing there is a hash function that maps keys to some values. But these hashing functions may lead to a collision that is two or more keys are mapped to same value. Chain hashing avoids collision. The idea is to make each cell of hash table point to a linked list of records that have same hash function value.
Hash Table Program in C - Online Tutorials Library
Hash Table Program in C - Hash Table is a data structure which stores data in an associative manner. In hash table, the data is stored in an array format where each data value has its own unique index value.
c - Implement a hash table - Stack Overflow
Oct 22, 2016 · I'm trying to create an efficient look-up table in C. I have an integer as a key and a variable length char* as the value. I've looked at uthash, but this requires a fixed length char* value.
Write a hash table in C - GitHub
Aug 23, 2017 · Hash tables are one of the most useful data structures. Their quick and scalable insert, search and delete make them relevant to a large number of computer science problems. In this tutorial, we implement an open-addressed, double-hashed hash table in C. By working through this tutorial, you will gain: